Unity: Introduction to the Core UI
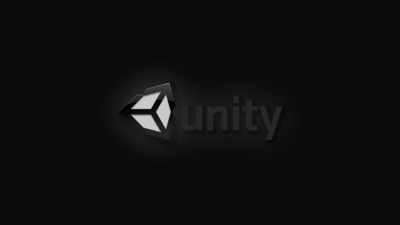
You could say I’m pretty green when it comes to knowing my way around the Unity game engine. Come with me on a journey through some core UI elements and a few silly examples, some of which may actually be practical in your next Unity project.
Update Unity Preference
I’m going to do you a real solid. Trust me, you’ll want to update the Playmode tint
to a really obnoxious color like #E70C6EFF
so that you know for a fact when you are in Play
mode. Props to @DJFariel for that tip.
This is something that drove me batshit crazy!
The Obligatory: Hello World
For this simple exercise we are going to use these three Core UI components:
- Visual UI: These are elements like Button, Image, Text, Toggle, etc.
- Rect Transform: Rect Transforms are reference points for anchoring, scaling, resizing, and rotation.
- Canvas: UI elements are children of a canvas. You can have multiple canvases in a single game scene. If you have no canvas but add some other UI GameObject, one will be automatically created and your UI GameObject will become a child of it.
Canvases have three different rendering modes:
- World Space: The canvas is a flat plane in the Camera scene, but the plane is relative to the camera’s orientation.
- Screen Space - Camera: The canvas is a flat plane and is always facing the camera.
- Screen Space - Overlay: UI elements are displayed without reference to a camera.
Since I’m three beers into this post already, I don’t really want to think in a 3-Dimensional space. So let’s render the canvas using Screen Space - Overlay mode.
In a new 2D project, let’s Add a Text
object to our scene. GameObject | UI | Text
and let’s name it _textHello
.
In the Inspector
perspective, let’s set the properties of _textHello
:
- Text =
Hello World
- Font =
Arial
- Font size =
35
- Alignment =
horizontal
andvertical center
- Horizontal and Vertical Overflow =
Overflow
- Color = Your choice.
Now let’s run the game and take a look at what we have done so far (notice the obnoxious pink color that overlays the Unity UI around the game scene).
Anybody Know What Time It Is?
Let’s build a digital clock. Add a Text
objects to our scene. GameObject | UI | Text
and let’s name it _textClock
.
In the Inspector
perspective, let’s set the properties of _textClock
:
- Text:
time will go here
- Font =
Arial
- Font size =
35
- Alignment =
horizontal
andvertical center
- Horizontal and Vertical Overflow =
Overflow
- Color = Your choice.
Also, we need to set the Rect Transform anchor to middle/center and the Pos Y
position to -60
.
We want the text of our clock to tell us the current time. To do this, we are going to write a C# script. Create a folder named Scripts
create a new C# script called DigitalClock
.
DigitalClock.cs
using System;
using UnityEngine;
using UnityEngine.UI;
public class DigitalClock : MonoBehaviour
{
private Text _clockText;
void Start ()
{
_clockText = GetComponent<Text>();
}
void Update () {
DateTime time = DateTime.Now;
string hour = LeadingZero(time.Hour);
string minute = LeadingZero(time.Minute);
string second = LeadingZero(time.Second);
_clockText.text = hour + ":" + minute + ":" + second;
}
string LeadingZero(int timeUnit)
{
return timeUnit.ToString().PadLeft(2, '0');
}
}
Now we’ll drag our DigitalClock
script to our _textClock
GameObject.
When you run the game, you can watch the clock update on the screen.
That’s pretty much a wrap on this basic tutorial there will be more to come on this later. I just got one question: